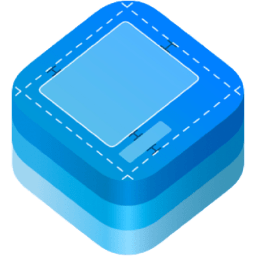
UIKit
23 posts
Working with diffable data sources and table views using UIKit
In this tutorial we're going to build a screen to allow single and multiple selections using diffable data source and a table view.
UIKit - loadView vs viewDidLoad
When to use these methods? Common questions and answers about the iOS view hierarchy including memory management.
10 little UIKit tips you should know
In this article I've gathered my top 10 favorite modern UIKit tips that I'd definitely want to know before I start my next project.
Custom views, input forms and mistakes
Just a little advice about creating custom view programmatically and the truth about why form building with collection views sucks.
Picking and playing videos in Swift
Learn how to record or select a video file using a video picker controller and the AVPlayer class, written entirely in Swift 5.
Building input forms for iOS apps
Learn how to build complex forms with my updated collection view view-model framework without the struggle using Swift.
Uniquely identifying views
Learn how to use string based UIView identifiers instead of tags. If you are tired of tagging views, check out these alternative solutions.
Styling by subclassing
Learn how to design and build reusable user interface elements by using custom view subclasses from the UIKit framework in Swift.
Picking images with UIImagePickerController in Swift 5
Learn how to get an image from the photo library or directly from the camera by using the UIImagePickerController class in Swift 5.
UITableView tutorial in Swift
This guide is made for beginners to learn the foundations of the UITableView class programmatically with auto layout in Swift.
Custom UIView subclass from a xib file
Do you want to learn how to load a xib file to create a custom view object? Well, this UIKit tutorial is just for you written in Swift.
UICollectionView data source and delegates programmatically
In this quick UIKit tutorial I'll show you how to create a simple UICollectionView without Interface Builder, but only using Swift.
Mastering iOS auto layout anchors programmatically from Swift
Looking for best practices of using layout anchors? Let's learn how to use the iOS autolayout system in the proper way using Swift.
UIColor best practices in Swift
Learn what are color models, how to convert hex values to UIColor and back, generate random colors, where to find beautiful palettes.
iOS custom transition tutorial in Swift
In this tutorial, you'll learn how to replace the push, pop and modal animations with custom transitions & percent driven interactions.
Ultimate UICollectionView guide with iOS examples written in Swift
Learn how to use UICollectionView, with highly reusable UIKit components and some MVVM pattern without the going nuts with index path calculations.
UICollectionView cells with circular images plus rotation support
Learn how to make rounded corners for UIImageView items wrapped inside collection view cells, with rotation support.
Self sizing cells with rotation support
How to make self sizing cells in Swift both for table & collection views supporting orientation changes and dynamic font types.
iOS Auto Layout tutorial programmatically
In this great iOS Auto Layout tutorial I'll teach you how to support rotation, use constraints, work with layers, animate corner radius.
How to create reusable views for modern collection views?
A quick intro to modern collection views using compositional layout, diffable data source and reusable view components.
The ultimate Combine framework tutorial in Swift
Get started with the brand new declarative Combine framework in practice using Swift. I'll teach you all the goodies from zero to hero.
Top 20 iOS libraries written in Swift
I gathered the best open source Swift frameworks on github that will help you to speed up mobile application development in 2019.
UIKit init patterns
Learn about the initialization process of the two well known classes in UIKit. Say hello to UIViewcontroller, and UIView init patterns.