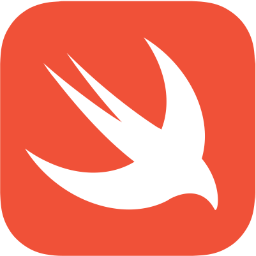
Swift
Swift is a powerful programming language by Apple, used for building both server-side applications and user interfaces with SwiftUI. Explore server-side frameworks like Vapor and Hummingbird, and learn best practices for creating robust backend services.
40 posts
Running tasks in parallel
Learn how to run tasks in parallel using the old-school tools and frameworks plus the new structured concurrency API in Swift.
Easy multipart file upload for Swift
Let me show you how to create HTTP requests using multipart (form data) body without a third party library. Simple solution.
Beginner's guide to modern generic programming in Swift
Learn the very basics about protocols, existentials, opaque types and how they are related to generic programming in Swift.
Async HTTP API clients in Swift
Learn how to communicate with API endpoints using the brand new SwiftHttp library, including async / await support.
Beginner's guide to Swift arrays
Learn how to manipulate arrays in Swift like a pro. This tutorial covers lots of useful array related methods, tips and tricks.
How to use a Swift library in C
In this tutorial, we're going to build a C app by importing a Swift library and talk a bit about the Swift / C Interoperability in general.
Building tree data structures in Swift
This tutorial is about showing the pros and cons of various Swift tree data structures using structs, enums and classes.
Practical guide to binary operations using the UInt8 type in Swift
Introduction to the basics of signed number representation and some practical binary operation examples in Swift using UInt8.
All about the Bool type in Swift
Learn everything about logical types and the Boolean algebra using the Swift programming language and some basic math.
How to build better command line apps and tools using Swift?
These tips will help you to create amazing CLI tools, utility apps, server side projects or terminal scripts using the Swift language.
Swift structured concurrency tutorial
Learn how to work with the Task object to perform asynchronous operations in a safe way using the new concurrency APIs in Swift.
Swift actors tutorial - a beginner's guide to thread safe concurrency
Learn how to use the brand new actor model to protect your application from unwanted data-races and memory issues.
Introduction to async/await in Swift
Beginners guide to the new async/await API's in Swift 5.5. Interacting with sync code, structured concurrency, async let.
Dynamic libraries and code replacements in Swift
How to load a dynamic library and use native method swizzling in Swift? This article is all about the magic behind SwiftUI previews.
Unsafe memory pointers in Swift
Learn how to use raw pointer references, interact with unsafe pointers and manually manage memory addresses in Swift.
Memory layout in Swift
Start learning about how Swift manages, stores and references various data types and objects using a memory safe approach.
How to use C libraries in Swift?
Learn how to use system libraries and call C code from Swift. Interoperability between the Swift language and C for beginners.
Building static and dynamic Swift libraries using the Swift compiler
This tutorial is all about emitting various Swift binaries without the Swift package manager, but only using the Swift compiler.
The Swift compiler for beginners
Learn how to build executable files using the swiftc command, meet the build pipeline, compilers and linkers under the hood.
Event-driven generic hooks for Swift
In this article I am going to show you how to implement a basic event processing system for your modular Swift application.
Getting started with SwiftIO
SwiftIO is an electronic circuit board that runs Swift on the bare metal. It can control sensors, displays, lights, motors and more.
Logging for beginners in Swift
Learn how to print variables to the debug console using different functions such as print, dump, NSLog and the unified os.log API.
How to define strings, use escaping sequences and interpolations?
As a beginner it can be hard to understand String interpolation and escaping sequences, in this tutorial I'll teach you the basics.
Building and loading dynamic libraries at runtime in Swift
Learn how to create a plugin system using dynamic libraries and the power of Swift, aka. modular frameworks on the server-side.
What's new in Swift 5.3?
Swift 5.3 is going to be an exciting new release. This post is a showcase of the latest Swift programming language features.
The Swift package manifest file
This article is a complete Swift Package Manager cheatsheet for the package manifest file, using the latest Swift 5.2 tools version.
How to download files with URLSession using Combine Publishers and Subscribers?
Learn how to load a remote image into an UIImageView asynchronously using URLSessionDownloadTask and the Combine framework in Swift.
Promises in Swift for beginners
Everything you ever wanted to know about futures and promises. The beginner's guide about asynchronous programming in Swift.
Beginners guide to functional Swift
The one and only tutorial that you'll ever need to learn higher order functions like: map, flatMap, compactMap, reduce, filter and more.
How to use the result type to handle errors in Swift 5?
From this tutorial you can learn how to utilize the do-try-catch syntax with the brand new result type to handle errors in Swift.
Swift 5 and ABI stability
Apple's Swift 5 language version will be a huge milestone for the developer community, let's see what are the possible benefits of it.
Generating random numbers in Swift
Learn everything what you'll ever need to generate random values in Swift using the latest methods and covering some old techniques.
Ultimate Grand Central Dispatch tutorial in Swift
Learn the principles of multi-threading with the GCD framework in Swift. Queues, tasks, groups everything you'll ever need I promise.
How to parse JSON in Swift using Codable protocol?
In this Swift tutorial, I'd like to give you an example about getting and parsing JSON data using URLSession and Codable protocol.
Deep dive into Swift frameworks
Learn everything about Swift modules, libraries, packages, closed source frameworks, command line tools and more.
How to call C code from Swift
Interacting with C libraries from the Swift language is really amazing, from this post can learn the most of C interoperability.
How to make a Swift framework?
Creating a Swift framework shouldn't be hard. This tutorial will help you making a universal framework for complex projects.
Swift enum all values
In this quick tutorial I'll show you how to get all the possible values for a Swift enum type with a generic solution written in Swift.
Everything about public and private Swift attributes
Have you ever heard about Swift language attributes? In this article I'm trying to gather all the @ annotations and their meanings.
Result builders in Swift
If you want to make a result builder in Swift, this article will help you to deal with the most common cases when creating a DSL.