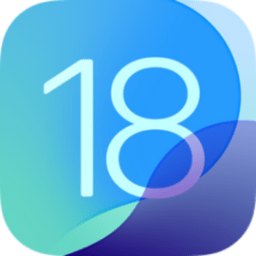
iOS
19 posts
Progressive Web Apps on iOS
This is a beginner's guide about creating PWAs for iOS including custom icons, splash screens, safe area and dark mode support.
What are the best practices to learn iOS / Swift in 2020?
Are you learning iOS development? Looking for Swift best practices? This is the right place to start your journey as a mobile application developer.
Picking and playing videos in Swift
Learn how to record or select a video file using a video picker controller and the AVPlayer class, written entirely in Swift 5.
URLSession and the Combine framework
Learn how to make HTTP requests and parse the response using the brand new Combine framework with foundation networking.
Uniquely identifying views
Learn how to use string based UIView identifiers instead of tags. If you are tired of tagging views, check out these alternative solutions.
Styling by subclassing
Learn how to design and build reusable user interface elements by using custom view subclasses from the UIKit framework in Swift.
Picking images with UIImagePickerController in Swift 5
Learn how to get an image from the photo library or directly from the camera by using the UIImagePickerController class in Swift 5.
Custom UIView subclass from a xib file
Do you want to learn how to load a xib file to create a custom view object? Well, this UIKit tutorial is just for you written in Swift.
UICollectionView data source and delegates programmatically
In this quick UIKit tutorial I'll show you how to create a simple UICollectionView without Interface Builder, but only using Swift.
Mastering iOS auto layout anchors programmatically from Swift
Looking for best practices of using layout anchors? Let's learn how to use the iOS autolayout system in the proper way using Swift.
How to use iCloud drive documents?
Learn how to sync files and data through a shared iCloud drive folder using the latest version of Swift programming language.
iOS custom transition tutorial in Swift
In this tutorial, you'll learn how to replace the push, pop and modal animations with custom transitions & percent driven interactions.
Networking examples for appleOS
Learn how to use Bonjour, with UDP/TCP sockets, streams and how to communicate through CoreBluetooth or the watch APIs.
UICollectionView cells with circular images plus rotation support
Learn how to make rounded corners for UIImageView items wrapped inside collection view cells, with rotation support.
Self sizing cells with rotation support
How to make self sizing cells in Swift both for table & collection views supporting orientation changes and dynamic font types.
iOS Auto Layout tutorial programmatically
In this great iOS Auto Layout tutorial I'll teach you how to support rotation, use constraints, work with layers, animate corner radius.
The ultimate Combine framework tutorial in Swift
Get started with the brand new declarative Combine framework in practice using Swift. I'll teach you all the goodies from zero to hero.
Top 20 iOS libraries written in Swift
I gathered the best open source Swift frameworks on github that will help you to speed up mobile application development in 2019.
UIKit init patterns
Learn about the initialization process of the two well known classes in UIKit. Say hello to UIViewcontroller, and UIView init patterns.